Lovable + Supabase how to fix: Application hangs up after user logs in
I have been using Lovable for about a week now to build two apps, both integrated with Supabase. In both cases, I encountered these exact two errors:
- The application hangs after a user logs in or when the page is refreshed with a logged-in user.
- Supabase error: "Infinite recursion detected in policy for relation."
Most Lovable users are non-developers, and even many developer users don’t thoroughly read the code Lovable generates. So unless you've encountered these errors before—either in previous projects or while using Lovable—they can be hard to spot.
In this article, I'll cover the first error.
This is also the beginning of a series where I'll address the second error and any other common issues I find when working with Lovable and Supabase.
Application hangs after login or page refresh with a logged-in user
In my first app, this error appeared after connecting Supabase and asking Lovable to create the full authentication flow, including a public profile for each user.
On the Supabase side, Lovable did a great job creating a profiles
table and setting up the correct trigger to generate a profile row when a user signs up. On the UI side, it also created all the required pages, and for the signup page, it included a couple of additional form fields that are later stored in the profiles
table.
All good, right? Well, not exactly—this change blocked the entire app!
After logging in with my test user or even refreshing the app, it froze. I asked Lovable to fix the issue, and its approach was to tweak the loading states of different pages. I attempted to fix it six times with prompting, but Lovable never identified the real problem.
Time to take the bull by the horns
One of the things I love about Lovable is its bidirectional integration with GitHub. So, I cloned the repository and started debugging the issue with the help of Windsurf.
Even then, Windsurf couldn’t detect the problem. But I remembered reading about this issue in the Supabase Discord help channel. After some searching, I found the post and noticed that Gary "The Goat" Abbott mentioned potential deadlocks in onAuthStateChange
.
So I checked the auth code. Can you spot the bug?
// Listen for auth changes
const { data: { subscription } } = supabase.auth.onAuthStateChange(
async (event, session) => {
if (session) {
await fetchProfile(session.user.id);
} else {
setSession(null);
setLoading(false);
}
}
);
Yes! The async callback! Lovable was making an asynchronous call to the profiles
table to fetch the user’s profile inside onAuthStateChange
. And according to the Supabase docs, this leads to deadlocks:
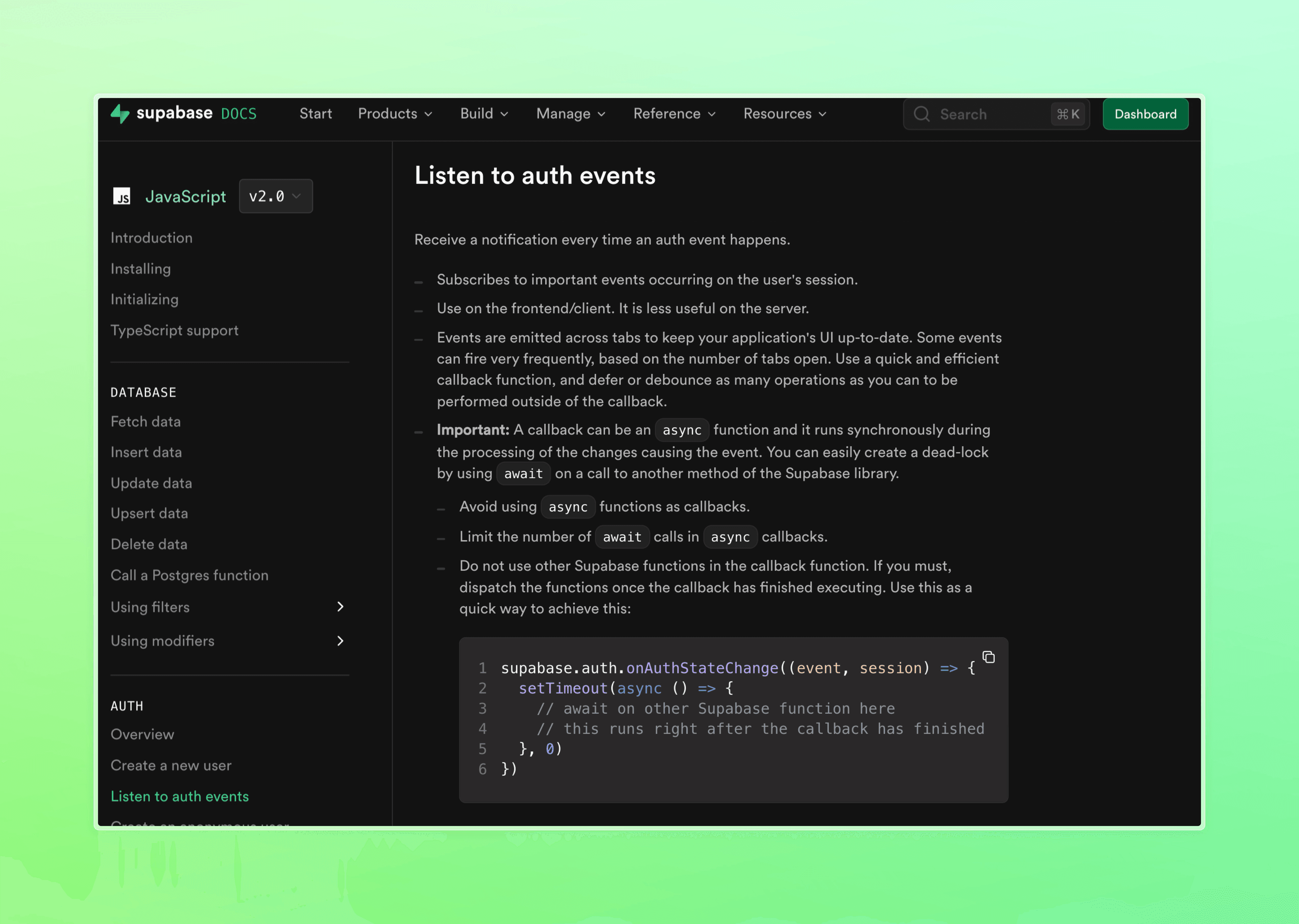
The fix is straightforward. If you're not a developer, you can simply copy and paste the warning from the Supabase docs into Lovable and ask it to resolve the issue in supabase.auth.onAuthStateChange
accordingly.
Fixing the issue in Lovable
This is a prompt example that you can copy and paste into Lovable:
Check every onAuthStateChange callback and make sure that they are not causing deadlocks by using async functions as recommended by Supabase:
Important: A callback can be an async function and it runs synchronously during the processing of the changes causing the event. You can easily create a dead-lock by using await on a call to another method of the Supabase library.
Avoid using async functions as callbacks.
Limit the number of await calls in async callbacks.
Do not use other Supabase functions in the callback function. If you must, dispatch the functions once the callback has finished executing. Use this as a quick way to achieve this:
supabase.auth.onAuthStateChange((event, session) => {
setTimeout(async () => {
// await on other Supabase function here
// this runs right after the callback has finished
}, 0)
})
Here's the fixed code Lovable generated:
// Set up auth state listener
const {
data: { subscription },
} = supabase.auth.onAuthStateChange((event, session) => {
setIsLoading(true);
if (session?.user) {
// Important: Use setTimeout to dispatch the fetch after the callback completes
// This avoids deadlocks as recommended in the Supabase docs
setTimeout(() => {
fetchUserProfile(session.user).finally(() => {
setIsLoading(false);
});
}, 0);
} else {
setUser(null);
setIsLoading(false);
}
});
No more app freezes after logging in or refreshing the page!
Preventing this issue in future Lovable apps
How can we ensure this doesn’t happen again when building a new Lovable app?
We can use Lovable’s project knowledge feature to explicitly define how it should handle onAuthStateChange
callbacks.
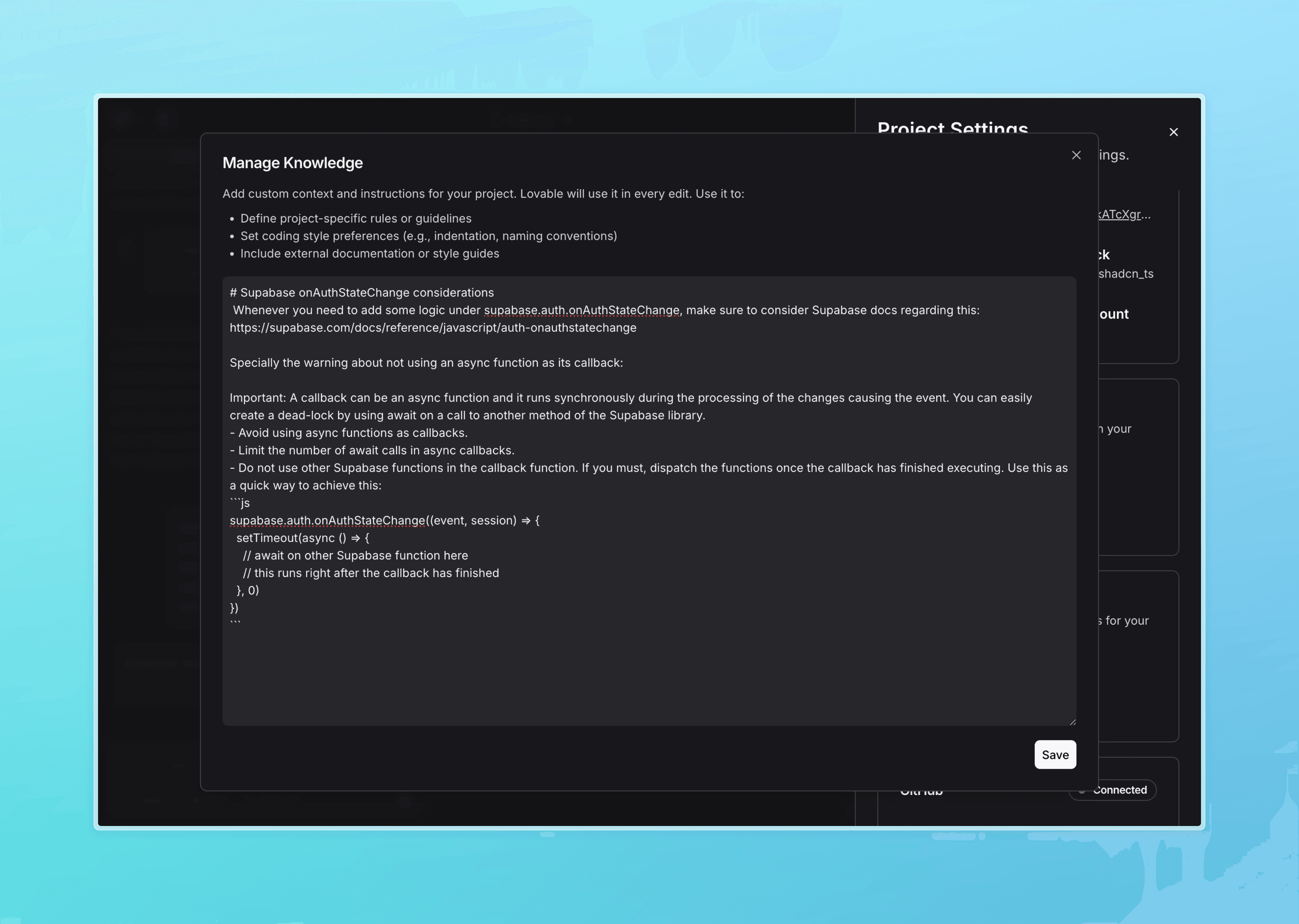
I tested a new project with this knowledge base entry, and Lovable successfully implemented onAuthStateChange
without falling into the same trap.
You can find a copy of this knowledge base in the this gist.
Note: Lovable tends to repeat the same mistake unless you explicitly document it in the project knowledge base. This is especially true when new code requires logic on auth state changes.
Final thoughts
It has been frustrating to see Lovable fail at such fundamental issues. Even more disappointing is watching users waste credits prompting Lovable to fix the issue — when it doesn’t even detect the root cause.
Hopefully, Lovable will retrain its models to better align with the Supabase documentation and avoid this and other issues in the future.
This is just the beginning of the series: Lovable + Supabase errors. I'll cover more common errors I find when working with Lovable and Supabase. So follow me on X for more troubleshooting tips.